Bash Compare Strings: How to Check if Two Strings Are Equal
Whether you’re validating user input or processing text data, knowing how to compare strings is an important skill.
In this blog post, we’ll look at four different methods you can use to check if two strings are equal. We’ll use examples to explain each method, providing you with a solid foundation for your future Bash scripting tasks. Let’s get started!
Prerequisite
To try out the scripts in this blog post, you need access to a Bash shell. You also need a text editor, such as "nano" or "vim", which come pre-installed by default in many Unix-like operating systems.
For the purpose of this blog post, I'll be using KodeKloud’s Ubuntu playground, which lets you access a pre-installed Ubuntu operating system in just one click. Best of all, you won't need to go through the hassle of installing any additional software— everything you need is already set up and ready to use.
Create a Script File
Let's start off by creating a script file. This is where we will be placing the scripts that we'll be writing in the upcoming sections.
To create a file named demo.sh
in the /usr/local/bin
directory, run the following command:
touch /usr/local/bin/demo.sh
Note: While you're free to create the demo.sh
file in any directory you prefer, we're placing it in the /usr/local/bin
directory for a specific reason. In most Linux distributions, this directory is included in the system's command path. This means we can run our script without making it executable.
Now that we have our script file set up, let's move on to the next section where we'll try out four different methods to check if two strings are equal.
Check if Two Strings Are Equal Using the test Command
The test
command is a built-in shell command used to evaluate conditional expressions. It has the following syntax:
test expression
Note: An expression refers to a condition that can be evaluated to either true or false.
Let's write a script that uses the test
command within an if/else structure to evaluate if two strings are equal. Here's the script:
#!/bin/bash
string1="KodeKloud"
string2="KodeKloud"
if test "$string1" = "$string2"
then
echo "Strings are equal"
else
echo "Strings are not equal"
fi
In this script, we first define two variables, string1
and string2
, and assign them the values KodeKloud
and KodeKloud
, respectively. We then use the test
command and the =
operator to compare these two strings. If the strings are equal, we print Strings are equal
. If they're not, we print Strings are not equal
.
Note that In Bash, you can either use =
(single equal sign) or ==
(double equal sign) for string comparison.
Before we can run this script, let’s place it inside the demo.sh
script file. To do this, open the script file in the "nano" text editor using the following command:
nano /usr/local/bin/demo.sh
Then place the script in the editor:
Now, save the file and exit the editor. In nano
, you can do this by pressing ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor.
It's time to run the script. You can do this by typing the following command:
bash demo.sh
After running this script, you'll see the output Strings are equal
, because the value of string1
(KodeKloud) is equal to the value of string2
(KodeKloud).
Note that string comparison is case-sensitive. To illustrate this, run the command nano /usr/local/bin/demo.sh
to open the script file in the "nano" text editor. Then convert the value of the string2
variable to all UPPERCASE (change KodeKloud to KODEKLOUD).
After making the changes, press ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor. Now, run the script with the following command:
bash demo.sh
You'll see that the output is Strings are not equal
, even though the letters are the same, because KodeKloud
and KODEKLOUD
are not equal in a case-sensitive comparison.
How can we then perform a case-insensitive match?
One way is to convert all the variable values to all lowercase (or all uppercase) before doing the comparison. Let’s apply this by converting the values of string1
and string2
variables to all lowercase. We can do so using the "${variable,,}"
syntax.
In this syntax, the variable name is followed by two commas. The double comma notation triggers the conversion of the variable's value to all lowercase.
Now, open the script file in the "nano" text editor using the command nano /usr/local/bin/demo.sh
. Then place the following script in the editor:
#!/bin/bash
string1="KodeKloud"
string2="KODEKLOUD"
string1="${string1,,}"
string2="${string2,,}"
if test "$string1" = "$string2"
then
echo "Strings are equal"
else
echo "Strings are not equal"
fi
Now, press ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor.
Finally, run the script with the following command:
bash demo.sh
You’ll see the following output:
This time, even though the original values of string1
and string2
had different cases, the output is Strings are equal
because we converted both variables to all lowercase before doing the comparison.
Check if Two Strings Are Equal Using the [ Command
The [
command is a built-in shell command, equivalent to the test
command. They both evaluate conditional expressions. The syntax of the [
command is as follows:
[ expression ]
An important point to note about this syntax is that there is a space before and after the square brackets. The ]
is actually the argument of the [
command.
Let's use this command in the script we wrote previously. Open your script file in the "nano" text editor by running the command nano /usr/local/bin/demo.sh
. Then replace the previous script with the following script:
#!/bin/bash
string1="KodeKloud"
string2="KodeKloud"
if [ "$string1" = "$string2" ]
then
echo "Strings are equal"
else
echo "Strings are not equal"
fi
As you can see, we've replaced test "$string1" = "$string2"
with [ "$string1" = "$string2" ]
.
After writing this script, press ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor. Now, run this script with the following command:
bash demo.sh
After running this script, you should see the output Strings are equal
, as shown below:
As we did in the previous section, if you want to perform a case-insensitive comparison, you can convert the variable values to all lowercase (or all uppercase).
Check if Two Strings Are Equal Using the [[ Keyword
The first thing to understand about [[
is that it's not a command; it's a keyword. If you run the command type [[
, you'll see that [[
is, in fact, a built-in shell keyword.
We use the [[
keyword the same way we use the [
command. We put the expression inside [[ ]]
. Here's the syntax:
[[ expression ]]
Let's use this [[
keyword in our script. Open your script file in the "nano" text editor by running the command nano /usr/local/bin/demo.sh
. Then replace the previous script with the following script:
#!/bin/bash
string1="KodeKloud"
string2="KodeKloud"
if [[ "$string1" = "$string2" ]]
then
echo "Strings are equal"
else
echo "Strings are not equal"
fi
As you can see, we've replaced [ "$string1" = "$string2" ]
with [[ "$string1" = "$string2" ]]
.
After writing this script, press ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor.
Now, run this script with the following command:
bash demo.sh
After running this script, you should see the output Strings are equal
, as shown below:
One of the benefits of the [[
keyword is that it supports case-insensitive comparisons when combined with the shopt
command's nocasematch
option.
In Bash, shopt
(stands for "shell options") is a built-in command used to toggle on and off the values of settings that control optional shell behavior. These shell options allow you to change the behavior of your Bash shell in a variety of ways.
Let's modify our script to utilize this feature. Open your script file in the "nano" text editor by running the command nano /usr/local/bin/demo.sh
. Then replace the previous script with the following script:
#!/bin/bash
shopt -s nocasematch
string1="KodeKloud"
string2="KODEKLOUD"
if [[ "$string1" = "$string2" ]]
then
echo "Strings are equal"
else
echo "Strings are not equal"
fi
shopt -u nocasematch
In our script, we're using shopt
with the -s
(set) flag and the nocasematch
option to make Bash pattern matching case-insensitive. At the end of the script, we return to case-sensitive behavior by unsetting the nocasematch
option with the -u
(unset) flag. This way, shopt
gives us a way to temporarily modify the behavior of the Bash shell to suit our needs, and then revert back to the original behavior when we're done.
After writing this script, press ctrl+o
to write the changes to the file, then press enter
to confirm the filename, and then press ctrl+x
to close the editor. Now, run this script with the following command:
bash demo.sh
After running this script, you should see the output Strings are equal
, as shown below:
Note: The shopt
command's nocasematch
option can only be used with the [[
keyword in Bash. It is not compatible with the test
command or the [
command.
Check if Two Strings Are Equal in One Line
In our previous discussions, we learned to check if two strings are equal using various shell commands, including test
, [
, and the built-in shell keyword [[
in multi-line scripts. Now, we can achieve the same results with just a single line of script.
First, let’s consider the test
command:
#!/bin/bash
test "KodeKloud" = "KodeKloud" && echo "Strings are equal." || echo "Strings are not equal."
Next, the [
command:
#!/bin/bash
[ "KodeKloud" = "KodeKloud" ] && echo "Strings are equal." || echo "Strings are not equal."
Lastly, the [[
keyword:
#!/bash/bin
[[ "KodeKloud" = "KodeKloud" ]] && echo "Strings are equal." || echo "Strings are not equal."
In each of these one-liner scripts, you'll notice we're using the &&
and ||
logical operators. These are known as the AND
and OR
operators, respectively.
The &&
symbol is a logical AND operator in Bash scripting, which means that if the condition before the &&
operator evaluates to true, only then will the command following it be executed. Conversely, the ||
symbol is a logical OR operator, which means that if the condition before ||
evaluates to false, only then will the command following it be executed.
Now, replace the previous script inside the demo.sh
file with these scripts and run them one by one. In each case, you will receive the following output:
These single-line scripts are particularly useful for quick operations where you need to execute a single command based on a condition. They make your script compact and concise. However, if your script needs to perform multiple operations based on the condition, it's more appropriate to use an if/else structure, which gives you the flexibility to include multiple commands.
Conclusion
In this blog post, we learned how to check if two strings are equal, mastering both case-sensitive and case-insensitive comparisons. We did this using the test
and [
commands, as well as the [[
keyword. We also learned how to carry out these equality string comparisons in a single line using the logical &&
and ||
operators.
Looking to build a solid foundation in shell scripting? Check out the Shell Scripts for Beginners course from KodeKloud.
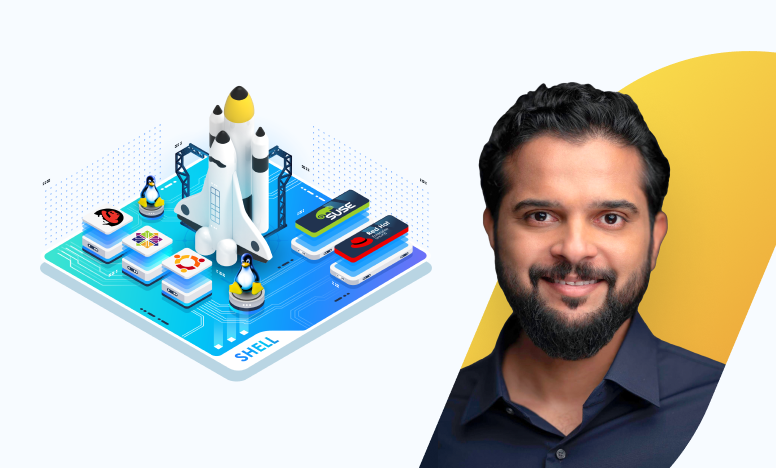
In this course, you'll dive into the practical world of Linux shell scripting. Regardless of your programming experience, you'll master fundamental scripting concepts such as variables, loops, and control logic. Throughout the course, you'll get plenty of hands-on experience using our comprehensive labs. Not only that, you'll also receive immediate feedback on your scripts, which will help you improve and refine them.
More Linux articles: