Terraform Input and Output Variables Explained
Terraform's input and output variables are powerful tools that enable developers to build flexible infrastructure configurations with Terraform.
Terraform is an infrastructure as code (IaC) tool used by developers to declaratively define and manage infrastructure resources. It offers an effective method for provisioning and managing cloud platforms alike. Terraform stands out as an IaC tool due to its input/output variable functionality that enhances the flexibility and modularity of infrastructure configurations.
In this article, we will explore Terraform input and output variables, their purpose, syntax, and use cases.
Understanding Input Variables
Terraform's input variables allow users to easily customize their configurations for specific environments or use cases, making them adaptable across environments or use cases. By taking advantage of input variables during deployment, values in the configuration files can easily be modified - an invaluable feature in building reusable and modular infrastructure configurations.
Declaring Input Variables
Below are the attributes you can set when declaring input variables:
- default - default value is used if no explicit value has been specified for a variable
- type - to declare the type of variable
- description - to state the purpose of the variable
- validation - to establish validation rules
- sensitive - Terraform makes use of context-sensitive variables; when set to "true", Terraform hides their value whenever displayed in any outputs
Input variables offer multiple data types. They are broadly separated into simple and complex data types. Simple data types are string, number, and bool, and complex data types are list, map, tuple, object, and sets or collections.
Try the Terraform Variables Lab for free
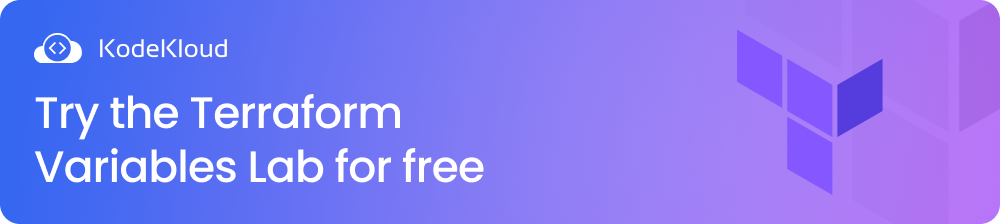
Input Variable Types
Below are some of Terraform’s variable types:
String
String variable type can store any text value. For instance, string variables are commonly used to represent geographic regions like, "us-west-1" or "eu-central-1". Below is an example that sets its region variable as a string type with a default value of "eu-central-1".
variable "region" {
type = string
default = "eu-central-1"
}
List
List variables allow users to sequentially pass multiple elements through Terraform configuration. As an example, you can create a list type variable for different instance types, as shown below:
variable "instance_types" {
type = list
default = ["c5.large", "r5.large", "c5.xlarge"]
}
Map
The "map" type of variable allows you to create variables with key-value pairs that you can add to your Terraform configuration as named values, and with each value you can associate a unique Key.
In the following example, the tags variable has been defined as a map type, more specifically, a map containing strings. By default, it has two key-value pairs. However, you can provide your own set of key-value pairs when using this variable.
variable "tags" {
type = map(string)
default = {
Name = "example-instance"
Environment = "staging"
}
}
Boolean
Bool variables can hold either true or false values, for instance, if we define enable_logging variables with the default value set to true then the logging function will be activated. You can change from true to false in order to disable it.
variable "enable_logging" {
type = bool
default = true
}
Using Input Variables
Once an input variable has been defined, its name can be referenced throughout your Terraform configuration using ${var.variable_name} syntax. For instance, say you want to set the region for an instance on Cloud Resources using Terraform, you could use an input variable like this:
variable "region" {
type = string
description = "The region where the infrastructure will be provisioned"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
region = var.region
}
In this example, the region input variable is used to set the region for AWS EC2 instances.
Assigning Default Values to Input Variables
Input variables may also have default values assigned, making them optional. You can accomplish this using the default field in a variable block; here's an example:
variable "region" {
type = string
description = "The region where the infrastructure will be provisioned"
default = "us-east-1"
}
Terraform will use the default values if no value is explicitly provided during deployment; any provided value overrides its default setting.
Assign Values to Input Variables
Terraform allows users to assign values to input variables in two ways; using a variables file or using parameters.
Assigning value to Terraform variables using a variable file
Input variable files are JSON or HCL files that contain key-value pairs for variables. To assign values using this method, we specify where to locate the variables file containing declared variables when running `terraform apply`. This is done by using the -var-file flag as shown below:
terraform apply -var-file="variables.tfvars"
In the command above, -var-file specifies the location of the variable file.
Assigning value to Terraform Variables using a parameter
We can also assign values using parameters declared when running `terraform apply`. We use a -var flag to add and declare a parameter, as shown below:
terraform apply -var="region=us-west-2"
This type of assignment can also be used to override values assigned to variables.
Understanding Output Variables
Output variables in Terraform serve as a means to expose specific data or insights about the provisioned infrastructure. These variables allow you to retrieve and utilize the information that is generated during the infrastructure deployment process. They are important for tasks such as retrieving IP addresses, DNS names, resource identifiers, or any other valuable data from the deployed infrastructure.
By capturing and sharing this information, output variables allow for data sharing between modules and coordination with external tools for automation purposes.
Declaring Output Variables
To declare an output variable in Terraform, you need to define it within a .tf file using the output block. Here's an example:
output "public_ip" {
value = aws_instance.example.public_ip
description = "The public IP address of the deployed instance"
}
In this example, we define an output variable named "public_ip" that references the public_ip attribute of an AWS EC2 instance named "example." The description field provides a description of the variable's purpose.
Using Output Variables
Once you have declared an output variable, you can reference it in other Terraform configurations using the output block's syntax. For instance, you can reference the public_ip output variable from another Terraform configuration file:
data "terraform_remote_state" "demo" {
backend = "s3"
config = {
bucket = "demo-bucket"
key = "demo.tfstate"
region = "us-west-2"
}
}
resource "aws_security_group_rule" "allow_ssh" {
type = "ingress"
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = [data.terraform_remote_state.example.public_ip]
}
In this example, we obtain the value of public_ip from a remote state and utilize it within a security group rule so as to only permit SSH access from that IP.
Sharing Output Variables
Terraform offers multiple methods for sharing output variables with external systems or components:
Command-Line Output: When running `terraform apply`, Terraform presents all output variables as command line output values, making it possible to easily view generated information.
Terraform State File: Output variables generated by Terraform are saved into its state file for later access by team members or external systems wishing to programmatically retrieve output variable values from it.
Data Sources: By making use of either the data block or terraform_remote_state data source, it's easy to extract output variables from remote state files or from other Terraform configurations.
Test your Terraform mastery with our free Terraform Challenges. They will help in polishing your infrastructure provisioning and management skills!
Conclusion
Terraform's input and output variables are powerful tools that enable developers to build flexible infrastructure configurations with Terraform. You can use input variables to parameterize configurations so they're adaptable across environments; output variables expose information from your infrastructure for reuse in other components or configurations. Mastering both can greatly increase modularity and maintainability, both of which are important to efficiently run infrastructure at scale.
Are you looking to polish your Terraform skills in a real-world environment? Enroll in our Terraform for Beginners Course, which covers all of Terraform fundamentals. It includes video lectures, interactive exercises, and hands-on labs to help you internalize concepts and commands.
If you want to gain other Infrastructure as Code (IaC) skills, check out our IaC Learning Path.