Terraform Variables: Types & Use Cases for Beginners
A variable is a container that holds a value. It can be used to represent different values at different times during the execution of a program. They can be assigned a value, which can then be changed or used in execution.
A variable in Terraform is similar to a variable in programming. It is a container that holds a value and can represent different values at different times during the execution of a Terraform plan. Understanding how to use variables in Terraform is essential to creating dynamic and reusable infrastructure as code.
This article will explore different types of terraform variables and how to use them.
What are Terraform Variables?
Terraform variables allow you to insert values in Terraform configuration files. They are used to store and manipulate data, such as IP addresses, usernames, and passwords. They can be assigned a value, which can then be changed or used as is in the configuration execution.
Terraform variables have multiple parameters that define how they behave. Below are some of the most commonly used parameters:
- type: Indicates the type of data that is to be stored in the variable. This parameter must be provided. Valid variable types include string, number, bool, list, etc.
- default: Sets the default value of variables. If no value is specified, this default value will be applied when Terraform commands run.
- description: This is an overview of the variable. This can be used to explain the purpose of a variable or its intended use.
- validation: You can create rules for validating variables. Information that is sensitive or confidential (such as passwords and keys of access) can be found in this section.
- nullable - Indicates whether a variable can be null within a module.
Terraform Input and Output Variables
Terraform uses input and output variable types to define and share values between different infrastructure configurations.
Try the Terraform Variables Lab for free
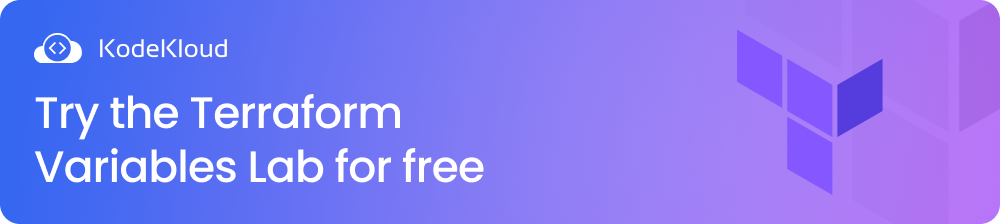
Input Variables
Input variables, created as variable blocks, can be used to parameterize Terraform configurations. You can use them to specify values for Terraform commands such as terraform plan or terraform apply. Here is a simple example of an input variable of type string.
variable "instance_type" {
description = "This is EC2 instance type"
type = string
default = "t2.micro"
}
Output Variables
We use Output variables to export values from a Terraform configuration file. They are defined within the output block in your Terraform configuration.
output "public_ip" {
description = "The public IP address of the EC2 instance"
value = aws_instance.example.public_ip
}
The terraform input variable types are divided into primitive and complex variable types.
Let us now look into these two types.
Primitive Variable Types
Below are the primitive variable types supported by Terraform:
- String
- Number
- Bool
String
String variable type can hold any text-based value. For example, string type can be used to represent a region, such as "us-west-1" or "eu-central-1". The example below defines the region variable as a string type with a default value of "us-west-2".
variable "region" {
type = string
default = "us-west-2"
}
Number
The number variable type can be an integer or a floating point value such as 2.5, etc. We can, for example, define the instance_count
variables to represent the number of instances deployed. In the example below, we defined instance_count
as a number with a default of 3.
variable "instance_count" {
type = number
default = 3
}
Bool
The Bool type is capable of holding either true or false values. For example, if we define the enable_logging
variables as a type of bool with a value defaulting to true, the logging function will be enabled. You can change the default value from true to false to disable it.
variable "enable_logging" {
type = bool
default = true
}
Complex Variable Types
Below are the complex terraform variable types:
List
List variables are used to sequentially pass multiple elements to Terraform configuration. As an example, you can create a list type variable for different instance types, as shown below:
variable "instance_types" {
type = list
default = ["t2.micro", "m4.large", "c5.xlarge"]
}
Map
The map variable type is used to create variables holding key-value pair pairs. This allows you to add a list of named values in your Terraform configuration. Each value will be associated with a unique Key.
In the following example, the tags
variable has been defined as a type of map, more specifically, a map containing strings. By default, it has two key-value pairs. However, you can provide your own set of key-value pairs when using this variable.
variable "tags" {
type = map(string)
default = {
Name = "demo-instance"
Environment = "production"
}
}
Object
The object variable allows you to create variables that contain structured data and named attributes. This is similar to a map but has fixed names and types for the attributes.
It is possible to define a user variable as an object type that has attributes such as name, age, and email. In the example below, the object has a default value of {"name" = "Sam Doe", "email" = "[email protected]", "age" = 30}
. It represents a user's name, email, and age.
variable "user" {
type = object({
name = string
email = string
age = number
})
default = {
name = "Sam"
email = "[email protected]"
age = 30
}
}
Set
Set variables allow you to define variables that hold an unordered set of unique values (i.e., no value is repeated). Sets can be useful when you don't care about the order and want to guarantee uniqueness.
For example, we can define a security_groups
variable as a set of strings.
variable "security_groups" {
type = set(string)
default = ["sg-12345678", "sg-abcdefgh"]
}
Tuple
The tuple variable type allows you to define an ordered collection of elements. For example, we can define the network_addresses
variable as a tuple of two strings.
variable "network_addresses" {
type = tuple([string, string])
default = ["192.168.1.1", "192.168.1.2"]
}
Both lists and tuples are used to store collections of data, but there is a key difference between them. Tuples are immutable, which means they cannot be changed once created. You cannot add, remove, or modify elements in a tuple. Whereas Lists are mutable, which means you can add, remove, or modify elements after the list is created.
What are Terraform Variable Definitions Files?
Terraform variable definitions files are files used in Terraform to declare and assign values to variables. These files provide a way to configure and parameterize Terraform configurations, allowing users to control various aspects of infrastructure provisioning and configuration dynamically. Variable definition files typically have the file extension .tfvars.
Note: Terraform variables can be defined in the infrastructure plan, but it's best practice to store them in a variable file.
Below is an example of a variable file named variables.tfvars.
variable "region" {
type = string
description = "The AWS region for the infrastructure"
default = "us-west-2"
}
variable "instance_type" {
type = string
description = "The EC2 instance type"
default = "t2.micro"
}
variable "subnet_ids" {
type = list(string)
description = "List of subnet IDs"
default = ["subnet-1", "subnet-2", "subnet-3"]
}
variable "num_instances" {
type = number
description = "The number of instances to create"
default = 1
}
The file above defines variables for subnet IDs, region, instance type, and the number of instances. The file also includes a description that gives information on the purpose of each variable.
How to define and use Terraform Variables?
Below are the three ways of defining variables in Terraform:
Command-line Flags: Pass variable values directly through command-line flags. For example:
terraform apply -var="region=us-east-1" -var="instance_type=t2.small"
Variable Files: You can store variables in separate files with a .tfvars extension. These files can contain multiple variable definitions and values. For instance, assume you have variables.tfvars file containing the code below:
variable "region" {
type = string
description = "The AWS region for the infrastructure"
default = "us-west-2"
}
variable "instance_type" {
type = string
description = "The EC2 instance type"
default = "t2.micro"
}
You can pass this variable file by running the terraform command below:
terraform apply -var-file=variables.tfvars
Environment Variables: You can set environment variables with a TF_VAR_ prefix to provide variable values. For example:
export TF_VAR_instance_type="t2.micro"
export TF_VAR_region="us-east-1"
Once you have defined your variables, you can reference them within your Terraform configuration files using the syntax var.<variable_name>. For example:
resource "aws_instance" "example" {
instance_type = var.instance_type
ami = "ami-0c55b159cbfafe1f0"
region = var.region
}
When you run terraform apply
or any other Terraform command, you'll be prompted to input values for any variables that haven't been defined or have been marked as required.
Conclusion
Terraform variables are a great way of simplifying the codebase and reusing values without hardcoding literal strings whenever you need to reference something. In this article, you have learned about different terraform variable types and how to use those variables.
Are you looking to polish your Terraform skills in a real-world environment? Enroll in our Terraform for Beginners Course, which covers all of Terraform fundamentals. It includes video lectures, interactive exercises, and hands-on labs to help you internalize concepts and commands.
To gain other Infrastructure as Code (IaC) skills, check out our IaC Learning Path.