What are Template Function and Pipeline in Helm?
When working with Helm, we’ll sometimes need to transform the data we supply as parameters into information that can be used during installation. For instance, converting values supplied in the .values file to strings that can be used in deployment creation. You might even need to chain several conversions together. Such a transformation is made possible by a template function and pipeline.
In this blog, we’ll explain what template functions and pipelines are and demonstrate how to use them. We'll start by creating a Helm Chart to demonstrate these two concepts.
Create a Helm Chart
Prerequisites: To follow along with the examples in this blog, you need to have a code editor and Helm installed on your local machine. Alternatively, you can use KodeKloud's Helm Playground. With this playground, you won't need to go through the hassle of installing any additional software— everything you need is already set up and ready to use.
Create a Chart
We create a Helm chart using the command helm create
. For instance, to create a chart named helm-charts, we use the command:
helm create helm-charts
This command creates a basic Helm chart that we can use to demonstrate how template functions and pipelines work. This command creates a directory named helm-charts. Within this directory is another directory named templates containing a deployment.yaml template - the file we'll use for our demo.
What is a Template Function?
A template function in Helm is a piece of code that can be used within a Helm template to manipulate or transform data. It is essentially a reusable block of code that can be called from different template parts to perform specific tasks. They can perform operations, such as formatting text, manipulating arrays and objects, and performing calculations.
Template functions allow developers to create dynamic and flexible templates for their Kubernetes deployments.
For instance, assume we've created a chart and assigned proper values to every customizable setting listed in values.yaml and referenced in deployment.yaml. For a line like image: "{{ .Values.image.repository }}:{{ .Values.image.tag }}"
, Helm can fetch the values we supplied in our values.yaml file and generate the final result, which might look something like image: "nginx:1.16.0"
.
Using functions in a chart is straightforward. Open deployment.yaml template file and navigate to the spec.template.spec.containers section. Here is a snippet of what it looks like:
containers:
- name: {{ .Chart.Name }}
In the file above, the container’s name is generated by {{ .Chart.Name }}
. This means the container takes the name helm-charts. You can verify this by checking the template using this command:
helm template ./helm-charts
Below is a screenshot of the container name in the generated manifest:
But what if this name has to respect some convention? For instance, if we need the container’s name to be in snake case, which uses an underscore "_" instead of a dash "-". We can use the snakecase
function to take the chart name helm-chart and transform it to snake case helm_chart.
Replace the container's name, which is represented by {{ .Chart.Name }}
, with {{ snakecase .Chart.Name }}
. Check the manifest using the command:
helm template ./helm-charts
Below is a screenshot showing the new container name:
From the file above, the container's name is in snake case now.
Generally speaking, you should think about functions whenever you need to transform the data you are fetching to your chart. However, it’s not limited to this scenario as there are many kinds of functions, and some can even generate data from nothing.
For example, if you want to add a timestamp to an object you generate in Kubernetes, you can use the now
function to add the current datetime to any location in the manifest. Here is a snippet showing how to implement the now
function:
timeLaunched: {{ now }}
The manifest generated by this chart would look like this:
timeLaunched: 2021-06-08 08:38:50.701447903 -0400 EDT m=+0.049411442
There are many more supported functions; you can see the full list here.
Sometimes, a single data transformation using one function isn't enough to achieve the desired result. For instance, when you want to extract a string and then transform the extracted string. This is where pipelines come in.
How to Use Pipelines in Helm Chart Templates
Pipelines are a powerful tool for connecting multiple functions together to perform chained tasks. Pipes allow the output of one function to be used as the input for another function, making it possible to create seamless workflows.
To create a pipeline, you use the vertical bar character (|) to connect two or more functions together. Pipelines in Helm charts are pretty similar to pipelines you might have seen or used in a Linux environment’s command line.
For example, to print abcd on the screen, we would use a simple command:
echo "abcd"
But if we want to take the output of this command and then process it in some way, we can use a pipeline in another command. In this case, we take the normal output of the echo
command - which would be abcd - pass it to the tr
command, which transforms all letters to uppercase, and then outputs the final result, as shown below:
user@debian:~$ echo "abcd" | tr a-z A-Z
ABCD
The syntax and logic for using pipelines in Helm charts are very similar.
Let’s open our deployment.yaml and see pipelines in action. First of all, let’s edit the line name: {{ snakecase .Chart.Name }}
. In this form, we use the function snakecase
, on the fetched value .Chart.Name
.
Let's create a pipeline that does the following on the name value:
- Fetches the chart name
- Add the word "Container" to the name
- Transform new full name to snakecase
- Convert the output into lowercase.
To perform these transformations, replace - name: {{ snakecase .Chart.Name }}
line with:
- name: {{ cat .Chart.Name "Container" | snakecase | lower }}
Below is a screenshot showing the new container name:
ENROLL in our Helm for Beginners course to dive deeper into these and more Helm concepts.
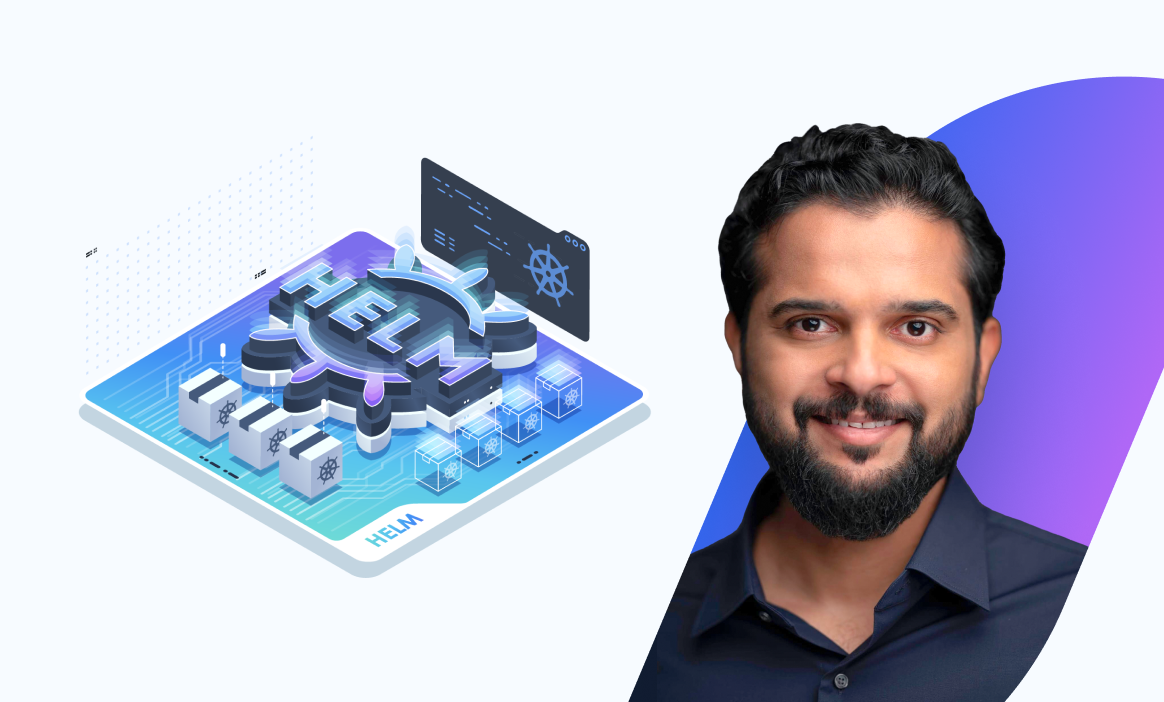
Best Practices When Using Functions in Helm Templates
When using functions and pipelines in Helm templates, following best practices is important to ensure your application is stable and maintainable. Here are some tips to keep in mind:
- Use built-in functions whenever possible. Helm provides many built-in functions that you can use to simplify your templates and reduce the risk of errors.
- Keep your functions and pipelines simple and easy to understand. Complex pipelines can be difficult to troubleshoot and maintain, so keep your logic as straightforward as possible.
- Use comments liberally. Comments can help you and other developers understand what your templates are doing and why.
- Test your templates thoroughly. Make sure that your templates work as expected before deploying them to production.
By following these best practices, you can create Helm templates that are easy to understand, maintain, and troubleshoot.
Step-by-step guide on how to install a WordPress website using Helm.
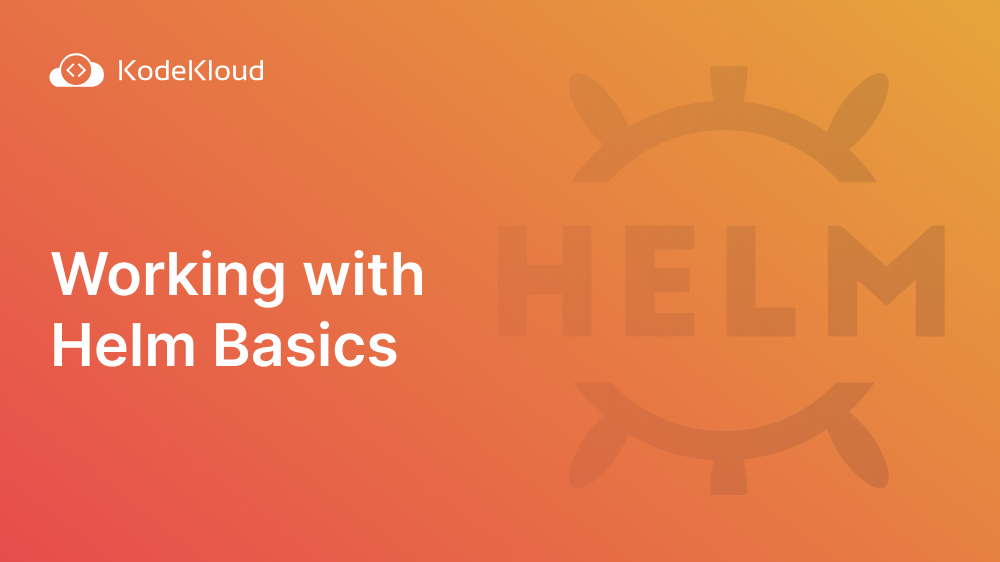
Conclusion
Functions allow you to perform various operations on your templates, such as manipulating strings or performing arithmetic operations. This can be incredibly useful when creating complex deployments with many different components. By understanding and using these functions effectively, you can create powerful and flexible Helm templates that can be easily deployed and managed.
Check out our Kubernetes Learning Path to better understand Kubernetes tools such as Linode, Kustomize, Lens, etc.
Gain real Kubernetes experience.
KodeKloud Engineer provides an environment for our students to gain real hands-on experience by working on real project tasks on real systems.
How it works
You will sign up as a System Administrator for xFusion Corp Industries - a fictional company created as part of the KodeKloud Engineer program. You will be given a project brief and access to the project documentation, architecture diagrams, and systems. Once ready, you will be assigned various tasks that are to be completed.
More on Helm: