How to Delete Local and Remote Branches in Git
You may have been in a situation where you need to switch between tasks you are working on, which are on different branches. But you probably don’t remember the full branch name you want to switch to. So, you run the git branch
command from your develop branch on your local machine but see a seemingly never-ending list of branches, which are primarily old feature branches that were completed and merged a while ago. Now, you decide to first do some branch cleanup before proceeding with your tasks.
There are several ways to go about deleting branches but before we dive into that, let’s first understand the inner workings of the different types of branches.
Key Takeaways
- To delete a local branch, which is not a remote-tracking branch, you need to switch to another branch to successfully perform the operation.
- Regularly deleting unused branches ensures you have a clean and organised repository, which enhances the collaboration experience.
- If you deleted a branch as an oversight, you can recover it by using the
git reflog
command.
What is a git branch?
A branch in Git is simply a pointer to a particular commit. There are 2 types of branches in Git:
- Local branch: a branch that is in your local repository on your local machine where you as a software engineer can develop a new feature, fix a bug or test your code changes.
- Remote branch: a branch that is on a remote repository that is on a remote server where you can either push your local branches to be code reviewed and merged or pull code changes from to ensure you have the latest changes.
Please note that the terms, local branch, and remote branch are not explicitly defined in Git. They are just concepts based on Git functionality. Also, you might have also heard of the main branch, develop branch, feature branch, release branch, or hotfix branch. These are actually popular branch naming conventions that are part of a popular branching strategy called Gitflow.
So, we can have a feature branch or a release branch for example, which are branches used in a gitflow branching strategy, that could either be a branch on your local machine (local branch) or a branch on a remote server (remote branch).
To LEARN how to connect Git with your CI/CD Pipeline, ENROLL in our Jenkins Course:
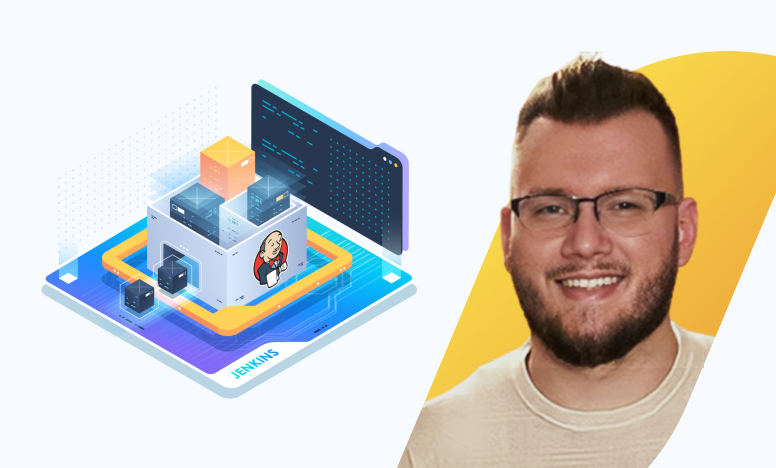
How to delete local git branches
Let’s go back to where we left off. Earlier, you had so many old feature branches completed some time ago but they are still on your local git repository and now you want to get rid of those old branches.
In the scenario above, your code changes have most likely been merged a while ago too. But there could be other scenarios where you have local git branches that have code changes that have not been merged yet into the current or active branch that you are on - remember that in this case, you are on the develop branch.
Now, this brings us to 2 scenarios:
- A local branch with merged code changes, where all your code changes have been merged into the current or active branch i.e. the develop branch.
- A local branch with unmerged code changes, where all your code changes have not yet been merged into the current or active branch i.e. the develop branch.
Let’s see how to delete your local branches for both scenarios mentioned above.
Delete a local branch with merged code changes
Below is the syntax for the command that deletes a local branch with merged code changes.
git branch -d <feature_branch_name_1> <feature_branch_name_n>
The example below demonstrates how to use the command:
git branch -d feature/a_new_feature feature/another_new_feature
The command above will delete the feature branches feature/a_new_feature and feature/another_new_feature. You can specify 1 or more branch names you want to delete.
The -d option is an alias for --delete, which will delete only specified branches whose code changes have all been merged into the branch you are on i.e. develop branch in this case.
Delete a local branch with unmerged code changes
Below is the syntax for the command that deletes a local branch with unmerged code changes.
git branch -D <feature_branch_name>
The example below demonstrates how to use the command:
git branch -D feature/a_new_feature feature/another_new_feature
The command above will delete the feature branches feature/a_new_feature and feature/another_new_feature. You can also specify 1 or more branch names that you want to delete.
The -D option is an alias for --delete --force i.e. -d -f, which will delete all specified branches regardless of whether the code changes have all been merged or not.
Please note that the git command git branch feature/a_new_feature -d -f
, similar to the one highlighted above, also deletes any specified branch whether or not it has merged or unmerged code changes.
How to delete remote git branches
Now you have successfully deleted the old feature branches on your local git repository. But then, you think the remote might also be cluttered with unnecessary branches which could be eating up storage space.
So, to satisfy your curiosity, you ran the command below:
git ls-remote --heads <remote_repo_alias>
The command above shows the branches that exist on the remote. The --head option ensures only branch references are listed. remote_repo_alias is an alias for the remote repository which can be called origin for example.
You see a couple of old feature branches in the output of the command above created by you that still exists on the remote. There are two methods you can use to delete such branches.
Method 1:
The first method’s command has the following syntax:
git push <remote_repo_alias> -d <remote_repo_branch_name>
The example below demonstrates how to use the command:
git push origin -d feature/a_new_feature
The command above will delete the remote branch feature/a_new_feature.
The -d option is an alias for --delete.
Method 2:
An alternative command for deleting a specified remote branch follows the syntax below:
git push <remote_repo_alias> :<remote_repo_branch_name>
The example below demonstrates how to use the command:
git push origin :feature/a_new_feature
In the example above, the origin is the alias given to the remote repo and feature/a-new-feature is the name of the feature branch you are deleting.
Another alternative to deleting a remote branch is via the remote repository’s (e.g. Github) web console by clicking the delete icon from the list of branches on the remote repository.
How to delete remote-tracking branches
A remote-tracking branch is a local reference to remote branches on the remote repository. They exist on your local git repository to track the state of associated remote branches. This kind of branch is automatically created in several ways. Some examples are:
- When you clone a remote repository via
git clone
. - When you download changes from a remote repository via
git pull
orgit fetch
. - When you push your local branch code changes to a remote repository via
git push
. - When you switch to a remote branch via
git switch
orgit checkout
, and so on.
The git branch -a
command gives you a list of your local branches and remote-tracking branches. -a is an alias for --all.
You can also run the git branch -r
command to see a list of only remote-tracking branches that exist on your local repository. -r is an alias for --remotes.
To delete a remote-tracking branch, you can use the same command used to delete a remote git branch. It also removes the associated remote-tracking branch which exists on your local repository.
Alternative ways to delete remote-tracking branches are seen below:
git remote prune <remote_repo_alias>
This command deletes remote-tracking branches on your local git repository for the specified remote i.e. remote_repo_alias which can be named origin for example.
git fetch <remote_repo_alias> --prune
This command fetches the latest updates (i.e new branches or commits ) from the remote specified by remote_repo_alias to update the associated remote-tracking branches and then deletes any stale remote-tracking branches on your local git repository which no longer exist on the specified remote. -p is an alias for --prune.
git branch -d -r <remote_repo_alias>/<remote_repo_branch_name>
This command is used to delete a specific remote-tracking branch from your local git repository.
Best practices when deleting branches
Below are some of the best practices to adhere to when deleting branches:
- You should periodically review and remove branches that have been merged already. This helps you keep a clutter-free repository.
- For important branches that have been merged and you don’t want to delete, you can back them up by archiving them.
- You should set up branch protection rules to avoid the accidental deletion of important branches. Remote repository platforms like Github, Bitbucket, and GitLab have this feature.
- Also, git hosting platforms like Github have a setting where you can automatically delete branches after they have been merged. This is another good option to avoid reviewing a very long list of remote branches and deciding which to keep or discard.
- Depending on the scenario, you should communicate with your colleagues before deleting a branch, especially one that is on the remote repository. This helps in ensuring that you don’t delete branches being used by other team members.
- You should periodically remove stale remote-tracking branches which no longer exist on the remote.
Benefits of deleting branches
Below are some of the benefits you get from deleting branches that are no longer being used:
- It helps keep your repository clean and organized by reducing clutter. It ensures that only the needed branches are maintained at every point in time which helps track progress on relevant branches.
- It helps in freeing up storage space, especially on your local machine. Branches consume disk space, hence deleting irrelevant branches helps you efficiently utilize your disk space.
- It improves network performance when running commands such as git fetch and git branch. This is as a result of the reduced repo size.
Common errors when deleting a branch and how to fix them
There are some errors you may see when you try to delete a branch. Let’s take a look at some of them.
Error deleting the same branch you switched to
error: Cannot delete branch 'feature_branch_name' checked out at 'C:/git/delete'
The error above occurs because, on your local git repository, you are trying to delete the same local branch you are currently on. Git will prevent you from performing this operation as it will result in a detached HEAD state. When you are in a detached HEAD state, you are not on any branch. This means any code changes you make will not be tracked and will be lost.
To resolve the error above,
- First, switch to another branch via
git switch
orgit checkout
- Then, you can go ahead to delete your local branches as discussed in the previous section.
Error deleting a branch with the same tag name
error: dst refspec feature_branch_name matches more than one.
error: failed to push some refs to 'https://remote_repository_url'
The error above happens because the feature_branch_name reference is the same name as another reference in the remote repository i.e. there are multiple references (e.g. branches, tags) in the remote repository with the same name you specified.
To resolve the error above, run the command below:
git push origin --delete refs/heads/<feature_branch_name>
This will delete the specified feature_branch_name from the remote repository specified as origin in the above example. Git uses refs/heads/ as a prefix to reference branches.
Alternatively, you can run the command below which does the same thing:
git push origin :refs/heads/<feature_branch_name>
In addition to the errors discussed above, you may have seen other errors depending on your use case. Feel free to comment below if you encounter any errors we have not covered in this article.
FAQ
Below is a frequently asked question about deleting branches:
What if you accidentally delete your local branch, is there a way to recover it?
You can use the git reflog
command to do so. The steps to do so are below:
- After the accidental deletion, run the command
git reflog
which lists all operations performed in your git repository e.g. deleting a branch, committing a change, reverting a change, etc., - From the output of the
git reflog
command, identify the commit hash or SHA1 associated with the latest commit on that branch, - Then, create a new branch using
git branch <branch_name> <commit_hash>
, which will now contain the branch code changes you accidentally lost.
Conclusion
Now you have a git repository that is clean and well organized. You will enjoy the benefit of running git operations, like git fetch, faster, especially for large repository sizes.
It is crucial to follow the best practices, particularly when collaborating with other team members, to ensure that only unnecessary branches are deleted.
More on Git: