How to Force Git Pull to Overwrite Local Files?
Imagine being so engrossed in working on a new feature on a software project and collaborating with a couple of teammates using Git. You have made some progress on a Feature Branch but have not staged or committed your work yet.
Now, you want to ensure that your feature branch gets all caught up with the Development Branch, as a few other teammates have pushed their feature branch to a Remote Repository and made a Pull Request (PR) which has been Code Reviewed, approved, and merged into the development branch. So, you do a git pull origin develop, but Oops, you get the error below:
error: Untracked working tree file 'some_file.go' would be overwritten by merge
You are probably experiencing deja vu at this moment, as this scenario seems familiar. You may have seen the above error or a variation of it, depending on your situation. So, what steps did you take to resolve this?
Was it the right approach for your use case? Did you feel there was probably a better and perhaps safer way to resolve this? Or did you end up writing all your code changes all over again because you lost them, which was not intended?
Let’s take a walk and get your questions answered!
Try the Git Pull Request Lab for free
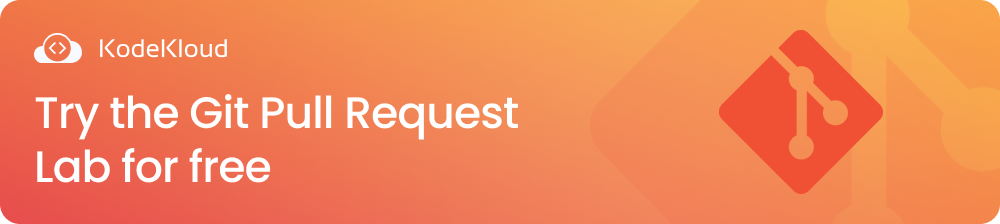
Key Takeaways
- Errors that have to do with overwriting local changes usually occur when you have uncommitted changes in your files that conflict with the same file changes from the remote repository.
- You can forcefully pull changes from a remote repository if you want to discard your local file changes.
- You can safely pull changes from a remote repository by first saving your local changes using a
commit
or astash
.
Why Did a Git Pull
Throw That Error Anyways?
To understand the git pull error, you need to know the types of git repositories.
Git is a distributed version control system. This means that there is a central remote repository, such as Github, used for collaboration with other developers on a project. On the other hand, each developer has their own Local Repository on their machine where they can make code changes before pushing them to the remote repository. This allows for a streamlined and efficient workflow when collaborating on projects with others.
Want a quick intro into Git and how to set it up in your system? Check out this video:
The local repository has three different stages: working directory, staging area, and commit history area. See the image below:
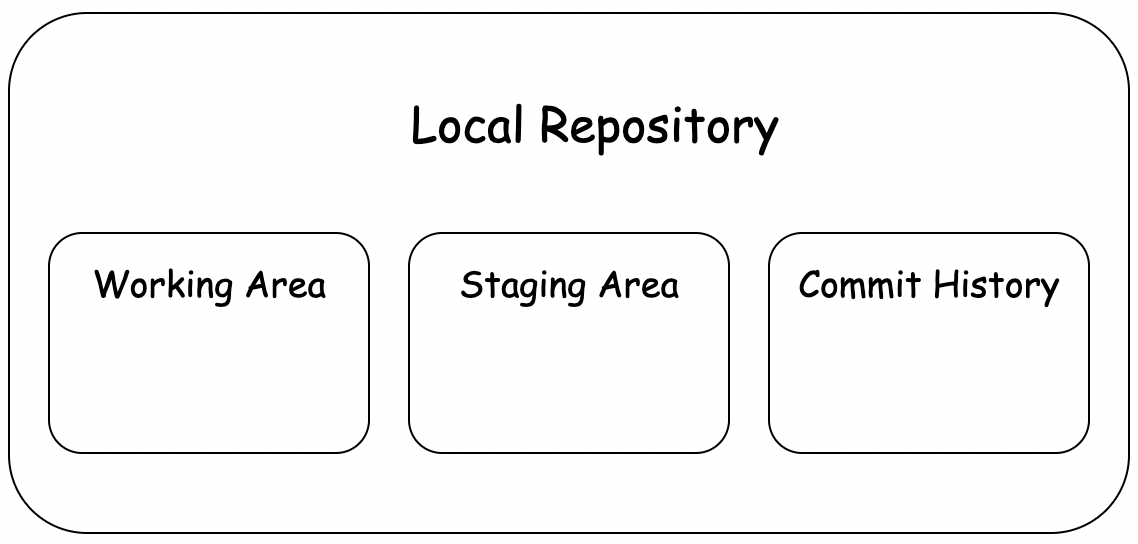
- The Working area or directory is where all your project files stay. Any file changes you make in your project are all in this area of your local repository, which is on your local machine. It contains Untracked changes that have not been staged or committed yet.
- The Staging area is where your project file changes that are ready to be committed stay. It contains Tracked changes that would be committed next.
- The Commit History area is the Git repository where all your commits are stored.
Now that you have understood the kinds of git repositories, let’s understand the error thrown.
What is This Error - “Untracked working tree file 'some_file.go' would be overwritten by merge”?
From the error message above, you have project file(s) in your Working area that have not yet been committed to the Git repository.
But you are trying to pull from a remote repository that most likely has file changes made on the same file(s) you are working on locally. So, Git threw that error to tell you that you would lose your local file changes, which conflicts with changes from the remote repository when you ran git pull.
The right solution to any problem ideally depends on the expected outcome, as each issue has its context and a desired outcome. So, depending on your scenario,
- You might not want to save the local file changes you have, and you are fine with Git overwriting them, or
- Perhaps, you don’t want to lose your file changes and want them saved first before pulling changes from a remote repository.
Let’s discuss both scenarios.
How to force git pull without saving local file changes
The approaches to be discussed here discard all your uncommitted local file changes by overwriting them. Let’s dive in.
Git pull <remote_repo_alias> <remote_repo_branch_name> --force
git pull origin develop --force
This will fetch changes from the remote repository aliased with 'origin' and merge them into your feature branch, disregarding any conflicting local changes you have made by overwriting them with changes from the remote.
Git fetch --all && git reset --hard <remote_repo_alias>/<branch_name>
git fetch --all && git reset --hard origin/develop
The git fetch --all
command fetches or downloads changes from all remote repositories defined in your local repository and uses these changes to update only the remote-tracking branches in your local repository. Please note that this command does not modify your local branches.
The git reset --hard origin/develop
command makes your feature branch point to the latest commit of the remote repository’s 'develop' branch. The --hard
option ensures that git discards any local changes that have not been committed, i.e., the uncommitted local changes in the working area or staging area will be lost.
Together, these commands will update your local feature branch with the latest changes from the remote’s 'develop' branch.
Please note that there are several other combinations of git commands which achieve the same result of overwriting your local file changes without saving them.
How to force git pull but save local file changes
Remember, in this scenario, you are already on your feature branch locally with changes in your working area or directory that have not yet been staged, let alone committed.
You can either commit your local changes or stash them.
Commit Local Changes
git add .
git commit -m "Add commit message before merge here"
git pull origin develop
# If any merge conflicts happen, manually resolve them and do the below
git add .
git commit -m "Add merge commit message here"
The commands above do the following, respectively:
- Adds files in the current directory (.) to the Staging area
- Creates a new commit that is stored in the Local Git Repository
- Fetches the latest changes from the develop branch on the remote repository aliased as origin
- If there are merge conflicts, resolve the conflicts manually. Then, stage your changes and commit your changes as done previously with the
git add
andgit commit
commands.
Stash Local Changes
git stash --include-untracked
git pull origin develop
# If any merge conflicts happen, manually resolve them and do the below
git add .
git commit -m "Add merge commit message here"
# Put your saved local changes back in your working area
git stash apply
The commands above do the following, respectively:
- By default, the
git stash
command only saves changes to files that git tracks, i.e., files that have been staged or committed. But with the--include-untracked
option, changes made to files that are both tracked (i.e., files in the working directory) and untracked are saved. - Fetches the latest changes from the 'develop' branch on the remote repository aliased as origin.
- If there are merge conflicts, it gives the option of resolving the conflicts manually, then staging your changes. And committing them as done previously with the
git add
andgit commit
commands. - Applies your saved changes which are your most recent stash, to your Working area and still keep the stash applied for future use, i.e., it does not remove an applied stash from the list of stashes you have.
How to fix other similar git pull errors
Let's look at some common errors below.
Error during checkout or switch:
error: Your local changes to the following files would be overwritten by checkout:
some_file.go
Please commit your changes or stash them before you switch branches.
Aborting
The error above usually happens when you run the git checkout
or the git switch
command. You could be trying to switch to another branch or commit hash when you have local changes that have not been committed yet (changes in files in your Working area or changes in files that have been Staged) that conflict with changes in the branch or commit you are trying to switch to. Git throws the above error to tell you that your changes will be lost in the process.
To resolve this error:
- If you want to discard your file changes: you can run the
git checkout
or thegit switch
command with the--force
option. - If you don’t want to lose your file changes: you can follow the steps explained previously by either committing your changes or stashing your changes first before switching to another branch or commit.
Error when you have changes in your working area and staging area:
error: Your local changes to the following files would be overwritten by merge:
some_file.go
Please commit your changes or stash them before you merge.
Aborting
The error above occurs when you have changes in files in your Working area or in files that have been Staged that conflict with the changes in the remote repository you are trying to pull from.
To resolve this error, you can follow the same steps discussed previously, depending on whether you want to discard or save your local changes.
FAQ
Below is a question that's asked frequently when working with Git:
What is the difference between git pull and git fetch?
git pull
is a combination of git fetch
and git merge
.
git fetch
fetches or downloads changes or updates from a remote repository and then updates the remote-tracking branches in your local repository. Note that by default, it fetches from the remote repository specified as origin. To fetch from all the remote repositories defined in your local repository, specify the --all
option i.e. git fetch --all
.
git merge
joins or combines changes from another branch into your current or active branch.
So, the git pull
command behind the hood fetches the latest updates from a remote repository and automatically tries to merge those changes into your current branch. If merge conflicts occur, you would need to resolve them manually before proceeding.
Conclusion
When you forcefully pull code from a remote repository, it can lead to the loss of the code changes you just wrote, which is mostly an unintended outcome. It is generally recommended to use an approach that will allow you to review file changes and resolve conflicts that arise.
Unless you are completely sure that you want to discard your changes, avoid forcefully pulling changes from a remote repository.
Interested in learning Git with simple visualizations, animations and by solving lab challenges? Check out our Git for Beginners Course