How to Uncommit Last commit in Git (5 Scenarios)
Have you ever wished you could turn back time and undo some erroneous implementations or features on your application’s code? Well, if you are a Git user, you are in luck. Git is a wonderful tool that lets you do exactly that: undo your mistakes and restore your project to a previous state. Git is a version control system that helps you manage your code and collaborate with others.
In this article, you will learn what a Git commit is and how to restore to a previous version in Git, depending on what you want to achieve. By the end of this article, you will be able to fix your blunders and get your project back on track.
Key Takeaways
- To undo the act of committing, but keep your changes and staging intact, use
git reset --soft HEAD
. - To undo the act of committing and also unstage your changes, but keep your files intact, use
git reset HEAD
. - To undo everything, including discarding your changes and resetting your files to the previous commit, use
git reset --hard HEAD
. And to undo a commit that you have already pushed to a remote branch, usegit revert <commit_hash> --no-edit
.
What are Commits in Git?
Git allows you to create snapshots of your project called commits. Each commit records what files you have changed, added, or deleted and also includes a message that explains why you made the change.
Commits are like checkpoints. They help you keep track of your progress and let you go back to a previous level if you mess up or want to try something different. However, sometimes you may create a commit that you regret later.
For example, you may have committed a file that has a bug, contains sensitive data, or is too large to upload to GitHub.
Try the Git Remote Repository Lab for free:
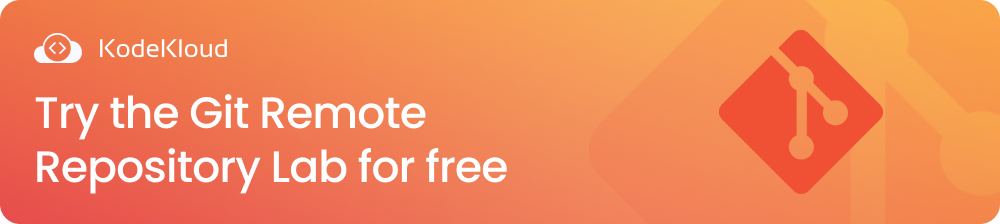
How to Uncommit Last commit in Git
In this section, we will discuss five different scenarios for undoing the last commit in Git; each with a different approach depending on whether you want to keep your changes and staging intact, completely remove the last commit and its changes or even revert your changes after pushing to a remote repository.
Scenario 1: Undo the act of committing, But Keep Your Changes and Staging Intact
Let’s say you have made some changes to your project and staged them using the git add
command. You are ready to commit them, so you type git commit -m "Added awesome feature"
and hit enter.
But then you realize that you forgot to do something important, like adding a comment or fixing a typo.
You want to undo your commit, but you don’t want to lose your changes or your staging. What do you do?
The answer is simple: use the git reset --soft HEAD~1
command. This command will undo your last commit, but it will keep your changes and your staging intact. It will move the HEAD pointer (which points to the latest commit on your current branch) to the previous commit. However, it will not touch the index (which stores the staged files) or the working tree (which contains the modified files).
In Git, the tilde symbol (~) is a shorthand notation used to refer to the parent commit of a particular commit.
For example, if you want to refer to the immediate parent commit of the current commit, you can use the tilde symbol followed by the number 1, like this: HEAD~1
. The 1 in this command specifies the number of steps or uncommits we want to do.
Here is an example of using this command. Suppose you have a project with two files: hello.py and README.md. You make some changes to both files and stage them using git add. Then you commit them using git commit -m "Added hello world"
. To see the history of your commits, run this command:
$ git log --oneline
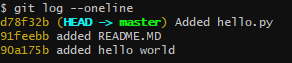
Now you realize that you made a mistake in hello.py, and you want to undo your commit. You can run the following command:
$ git reset --soft HEAD~1
Scenario 2: Undo the Act of Committing and Also Unstage Your Changes, But Keep Your Files Intact
Assume that you have made some changes to your project and committed them.
But then you realize that you don’t want to commit those changes at all. You want to undo your commit and also unstage your changes, but you don’t want to lose your files.
To do that, run the following command.
$ git reset HEAD~1
This command will undo your last commit and also unstage your changes, but it will keep your files intact. It will move the HEAD pointer to the previous commit, and it will also reset the index to match the HEAD.
However, it will not touch the working tree, which means your files will remain as they were before.
Scenario 3: Undo Everything, including Discarding Your Changes and Resetting Your Files to the Previous Commit
Assume you made some changes to your project, staged, and committed them. But then you realize that you made a huge mistake and want to undo everything. You want to discard your changes and reset your files to the previous commit.
To achieve that, run the following command:
$ git reset --hard HEAD~1
This command will undo your last commit and also discard your changes and reset your files to the previous commit. It will move the HEAD pointer to the previous commit, and it will also reset the index and the working tree to match the HEAD. This means that any changes you have made since the last commit will be erased permanently.
Here is an example:

This tells you that the current branch is now pointing to the commit with the hash 1a6fd59, which has the message Added hello.py
Scenario 4: Undo A Commit That You Have Already Pushed to A Remote Branch
Assume you made some changes to your project, staged, committed, and pushed them to a remote branch.
But then you realize that you don’t want to share those changes with others or merge them into the main branch. You want to undo your commit and remove it from the remote branch. To achieve that, run the following command:
$ git revert <commit_hash> --no-edit
This command will undo your commit by creating a new commit that reverses the changes of the previous commit. It will not delete or modify the previous commit, but it will apply the opposite changes to your files. Then you can push the new commit to the remote branch using git push origin main.
Suppose you still have a project with two files: hello.py and README.md. You make some changes to both files, then you push them to a remote branch. To see the history of your commits on both local and remote branches, you can run the following command:
$ git log --oneline --all --graph
Now you realize that you don’t want to share those changes with others or merge them to the main branch. You can use git revert <commit_hash> --no-edit
to undo your commit and create a new commit that reverses the changes of the previous commit. You need to specify the hash of the commit you want to undo, which is d78f32b in my case:

You can see that there is a new commit with the message “Revert "Added hello.py" that reverses the changes of the previous commit. You can also use git diff HEAD
to see what changes were made by the revert:
You can now push the new commit to the remote branch using git push <remote folder name> main
. To verify that the commit has been created, run the following command:
$ git log --oneline --all --graph
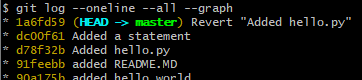
You can see that the branch has been updated with the new revert commit.
The Advantages of This Approach
This approach offers several advantages
- You can undo your last commit and remove it from the remote branch without deleting or modifying it.
- You can create a new commit that reverses the changes of the previous commit and keep your history consistent.
- You can share your revert with others and avoid merging unwanted changes to the main branch.
The Drawbacks of This Approach
Here's the drawback of using this approach
- You can only undo one commit at a time. If you want to undo multiple commits, you have to repeat the command.
- You have to use the commit hash to specify which commit you want to undo. You can use
git log --oneline
to find the hash or use a relative reference like HEAD^ to refer to the last commit.
Scenario 5: Undo Several Commits and Move More Than One Step Back
Sometimes, it's not enough to simply undo your most recent commits; you might find yourself needing to revert back more than just one commit. Suppose you’re using the git reset --soft HEAD
command, but you wish to go back three commits.
To achieve this, run the following command, replacing N with the number of uncommits you want to perform:
$ git reset --soft HEAD~N
In this case, the command would be:
$ git reset --soft HEAD~3
This command moves the HEAD pointer back by 3 commits while keeping your changes and staging intact.
After running the command, you will be positioned at the commit you specified, with the changes from the subsequent commits unstaged.
Tips and Best Practices to Avoid or Minimize Mistakes In Git
It is important to be careful when using Git commands, as some of them can overwrite or erase your work permanently.
Below are tips that will minimize your mistakes in Git.
- Before committing anything, always check the status of your repository using
git status
and review the changes you have made usinggit diff
orgit diff --staged
. - Before pushing anything to a remote branch, always check the history of your commits using
git log --oneline
orgit log --oneline --all --graph
and make sure that everything is as expected. - Before using any destructive commands like
git reset --hard
orgit push --force
, always make a backup or a copy of your repository somewhere else. You can also usegit reflog
to see the history of your actions and recover lost commits. - Use meaningful and descriptive commit messages that explain what and why you have changed something. Avoid using vague or generic messages like “Update files” or “Fix bug.”
- Use branches and pull requests to organize your work and collaborate with others. Avoid working directly on the main branch or pushing untested or unfinished code to a remote branch.
Also, check out the Git cheat sheet for more information.
Conclusion
In this article, you have learned how to uncommit your last commit in Git, depending on what you want to achieve. You have also seen how to uncommit several commits at once. To make the most out of Git, ensure you adhere to the best practices discussed in the previous section.
To learn more about Git’s basic concepts, check out this video:
Interested in learning more about Git? Check out the GIT for Beginners course. It covers how to get started with Git, useful Git commands, the internals of Git, and how it’s actually working under the hood. It also comes with hands-on labs to help you internalize concepts and commands covered in each lecture.