Git Switch vs. Checkout: What’s the Difference?
One of the most important features of git is the ability to create and work on different branches. Branches are like parallel timelines of your project, where you can work on different features or experiments without affecting the stable code. We navigate between branches using either git switch or git checkout.
Want a quick intro to Git and how to set it up in your system? Check out this video:
Git Basics for Devops Beginners
This article will explain the syntax and usage of git switch and git checkout, provide some usage examples, and compare the two commands side-by-side. Lastly, we’ll discuss when to use each.
Key Takeaways
- Git switch and git checkout are both used to change branches, but they have some differences in syntax and behavior. Git switch is a newer command that is more focused on branches, while git checkout is an older command that can do other things besides switching branches.
- Git checkout has a more versatile syntax than git switch, but it can also be more confusing and error-prone if you don’t understand its behavior.
Try the Git Branches Lab for free
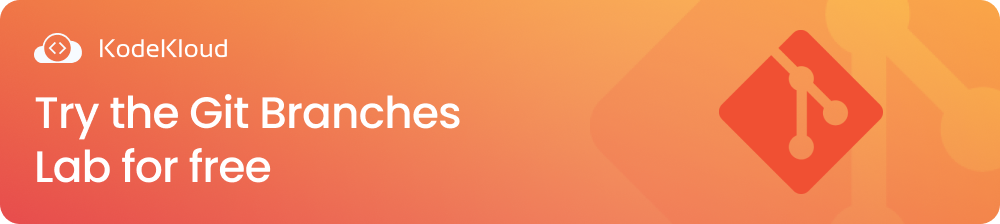
Git Checkout
git checkout is a versatile command used to navigate between branches, restore files, create new branches, and update submodules. In this article, we will focus on its use in navigating between branches. Below is the command’s branch switch syntax:
git checkout <branch>
This command will change your current branch to the one specified by <branch>. Below are some of the command’s additional options that can modify its behavior:
- The -b or --branch option will create a new branch with the given name and switch to it. This is equivalent to using git switch -c.
- The -B or --force-create option will create or reset a branch with the given name and switch to it. This can be useful if you want to overwrite an existing branch with a different commit or branch.
- The -t or --track option will create a new branch that tracks a remote branch with the same name and switch to it. This is equivalent to using git switch without specifying a branch name.
- The -f or --force option will discard any uncommitted changes in your working directory and staging area before switching. This is equivalent to using git switch -f.
Git Checkout Usage With Examples
Switch to an existing branch
If you want to switch to an existing branch called feature1, you can simply run:
git checkout feature1
Create a new branch based on current branch
If you want to create a new branch called feature2 based on the current branch and switch to it, you can run:
git checkout -b feature2
Create or reset a branch and switch to it
If you want to create or reset a branch called feature3 based on master and switch to it, you can run:
git checkout -B feature3 master
Create a new branch that tracks a remote branch and switch to it
If you want to create a new branch that tracks a remote branch called feature4 and switch to it, you can run:
git checkout -t feature4
Force-switch to another branch and discard any uncommitted changes
If you want to force-switch to another branch called feature5 and discard any uncommitted changes in your working directory and staging area, you can run:
git checkout -f feature5
The Advantages Of Using Git Checkout
Here are the advantages of using git checkout:
- It can do more things than just switching branches.
- It works with older versions of git.
The Disadvantages Of Using Git Checkout
Here are the disadvantages of using git checkout:
- It is more ambiguous and confusing since it can do many things besides switching branches.
- It can be dangerous if you use it without understanding what it does. You might accidentally overwrite or lose your changes or branches.
Git Switch
The git switch command was introduced in Git 2.23 as a way to split and clarify the two different uses of git checkout: switching branches and restoring files. The idea behind this move is to let people use git switch for switching branches and git restore for undoing changes from a commit.
The git switch command can only be used for branch creation and switching. It has a simpler syntax and fewer options than git checkout. Below is its syntax:
git switch <branch>
This command will change your current branch to the one specified by <branch>. If the branch does not exist locally, it will try to find it on the remote repository and create a local copy. If the branch name is ambiguous (for example, if there is a local branch and a remote branch with the same name), it will ask you to specify which one you want.
The git switch command also has some options that can modify its behavior
- The -c or --create option will create a new branch with the given name and switch to it.
- The -d or --detach option will switch to a specific commit without creating a new branch. This is useful for testing or debugging purposes, but be careful not to lose your changes when you switch back to a normal branch.
- The -m or --merge option will try to merge your current branch into the new one before switching. This can help you avoid conflicts or keep your changes in sync.
- The -f or --force option will discard any uncommitted changes in your working directory and staging area before switching. This can be dangerous if you have important changes that you haven’t saved yet.
Git Switch Usage With Examples
Switch to an existing branch
If you want to switch to an existing branch called feature1, you can simply run:
git switch feature1
Create a new branch and switch to it
If you want to create a new branch called feature2 based on the current branch and switch to it, you can run:
git switch -c feature2
Switch to a specific commit without creating a new branch
If you want to switch to a specific commit with the hash 1234567 without creating a new branch, you can run:
git switch --detach 1234567
Merge current branch into master before switching to it
If you want to merge your current branch into master before switching to it, you can run:
git switch -m master
Force-switch to another branch and discard any uncommitted changes
If you want to force-switch to another branch called feature3 and discard any uncommitted changes in your working directory and staging area, you can run:
git switch -f feature3
The Advantages Of Using Git Switch
Here are some of the advantages of git switch:
- It is more intuitive and clearer than git checkout since it only deals with switching branches.
- It has some useful options that can help you create, merge, or detach branches easily.
- It can automatically fetch remote branches if they don’t exist locally.
The Disadvantages Of Using Git Switch
Though the git switch is less ambiguous and more intuitive, it still has some drawbacks. Here are some of them:
- It is relatively new and not available in older versions of git. You might need to update your git version or use an alternative command if you work with older repositories or systems.
- It can be confusing if you are used to using git checkout for switching branches. You might need to adjust your habits or remember the differences between the two commands.
Tips And Best Practices For Choosing Between Switch And Checkout
Here are some tips and best practices for choosing between switch and checkout:
- Always make sure your working directory has no unstaged changes and staging area has no uncommitted changes before switching branches. You can use git status to check for any uncommitted changes or conflicts. You can also use git stash to save your changes temporarily and restore them later.
- Always update your local repository before switching branches. You can use git fetch or git pull to get the latest changes from the remote repository. This will help you avoid errors or conflicts when switching branches.
- Always specify the branch name when switching branches. This will help you avoid ambiguity or confusion when using either command. You can also use tab completion or git branch -a to see the available branches.
- Always be careful when using the force option (-f or --force) with either command. This will discard any uncommitted changes in your working directory and staging area before switching branches. Make sure you don’t have any important changes that you haven’t saved yet.
Conclusion
While both git switch and git checkout can be used to navigate between branches in Git, they have some subtle differences. git switch mainly deals with switching branches and has some useful options that can help you create, merge, or detach branches easily. Git checkout can do many things besides switching branches, such as restoring files from previous commits, creating new branches, or updating submodules.
The choice between the two commands depends on your preference and situation. You might prefer to use git switch if you want a simpler and safer way to switch branches or if you work with newer versions of git. You might prefer to use git checkout if you want a more versatile way to switch branches or if you work with older versions of git.
Interested in learning Git with simple visualisations, animations and by solving lab challenges, check out our Git for Beginners Course.