How to Add YAML Comments with Examples
Comments are an essential part of code because they help explain what the code is doing. They also help make the code clean, organized, and easily understandable.
In this article, we’ll look at how to add comments in YAML and the different use cases. Additionally, we’ll discuss the best practices to follow when implementing it.
What Is Yaml?
YAML is a human-readable data-serialization language mainly used to write configuration files. Unlike JSON or XML format, YAML is designed for readability, allowing even non-technical people to understand what the code does. YAML supports many data types, from strings, numbers, boolean, dates, timestamps, and arrays to maps. For these reasons, platforms like Kubernetes, Ansible, and GitLab CI use YAML as their scripting language.
How To Add Comments in Yaml
Comments in YAML are denoted by the "#" symbol and are ignored by the interpreter when the code is executed. We add the symbol at the position we want the comment to start. If you add this symbol in front of code characters, the interpreter will treat that code after the symbol and in that line as comments.
Try the Kubernetes Deployments Lab for free
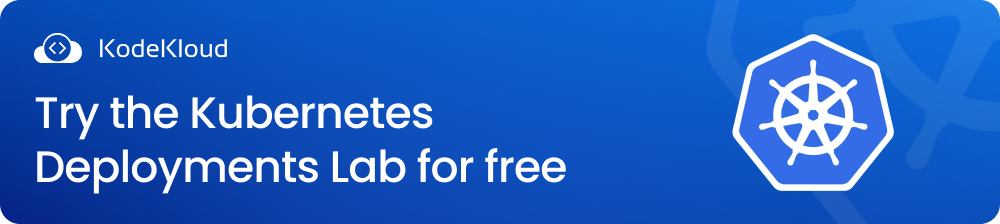
How to Add Single Line Comment in YAML
Below is an example of how to add a single-line comment in YAML:
apiVersion: v1
kind: Secret
#Specifying the name of the deployment
metadata:
name: mysql-root-pass
type: Opaque
stringData:
password: mysql123
In the code above, the comment, ‘Specifying the name of the deployment,’ is ignored by the interpreter.
How to Handle Multiple Line Comments in YAML
YAML does not have in-built support for multi-line comments, but there is a way to handle such comments. Suppose you want to write comments for multiple lines. In that case, you need to add a “#” symbol at the beginning of every line, as shown below:
apiVersion: v1
kind: Secret
#Specifying the name of the deployment
#You can use this name to reference the deployment object
metadata:
name: mysql-root-pass
type: Opaque
stringData:
password: mysql123
From the code above, you see that creating multiple-line comments requires the creation of consecutive single-line comments.
Comments Can Be In-Line
A YAML comment does not necessarily need to be in a line separate from the code. You can also add it in the same line with your code. Below is an example of how an in-line comment works:
apiVersion: tekton.dev/v1beta1
kind: Task
metadata:
name: hello-task
spec:
steps:
- name: hello
image: ubuntu
script: > # Define the commands you want to run in this script
echo "Hello"
In the example above, the interpreter will treat the characters after ‘#’ and in that line as comments.
Yaml Does Not Support Comments Inside “String”
YAML comments do not work inside the “string”. If you put “#” inside the string, YAML will consider it regular text and not mark the rest of the line after the “#” character as comments. Take the Tekton Task definition below:
apiVersion: tekton.dev/v1beta1
kind: Task
metadata:
name: hello-task
spec:
steps:
- name: hello
image: ubuntu
script: >
echo "# is just a regular text"
In this example, the echo command will print to the console, “# is just a regular text”.
Comments Must Not Be Inside the Block Scalars
In YAML, scalars refer to primitive data types like strings, booleans, integers, or floats. A block scalar in YAML is denoted by a pipe “|” or greater than “>” symbol. Below is an example of a block scalar in YAML:
summary: block scalar
description: |
This is my sentence with the literal scalar.
And another sentence.
operation: delta
YAML does not support comments inside the block scalars. If you add “#” inside the block scalar above, YAML will consider it part of the multi-line strings. See the example below:
summary: block scalar
description: |
# This is my sentence with the literal scalar.
And another sentence.
operation: delta
The description value for this YAML file will be: “# This is my sentence with the literal scalar. And another sentence.”
Why Add Comments in Yaml
Below are some of the reasons why we add comments in YAML:
Document the Usage of the Yaml Code
When defining software deployment files, you need to include a lot of details. Adding comments lets you and other developers know what each part of the script is intended to do. This makes the code readable, maintainable, and less prone to errors.
Let’s look at an example of this. Below is a well-commented Kubernetes role definition file:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: tekton-triggers-minimal
namespace: tekton
rules:
# EventListeners need to be able to fetch all namespaced resources
- apiGroups: ["triggers.tekton.dev"]
resources: ["eventlisteners", "triggerbindings", "triggertemplates", "triggers", "interceptors"]
verbs: ["get", "list", "watch"]
- apiGroups: [""]
# configmaps is needed for updating logging config
resources: ["configmaps"]
verbs: ["get", "list", "watch"]
# Permissions to create resources in associated TriggerTemplates
- apiGroups: ["tekton.dev"]
resources: ["pipelineruns", "pipelineresources", "taskruns"]
verbs: ["create"]
- apiGroups: [""]
resources: ["serviceaccounts"]
verbs: ["impersonate"]
- apiGroups: ["policy"]
resources: ["podsecuritypolicies"]
resourceNames: ["tekton-triggers"]
verbs: ["use"]
The code above creates a new Kubernetes role with several permissions so Tekton can access the Kubernetes components to deploy the application.
If, in the future, another developer looks at an uncommented permission list, it will be difficult to understand all the components. They will use more time trying to understand it than they should - resulting in a slower application deployment process. Additionally, they might misunderstand the permissions in the script and make changes that cause a system failure.
Adding comments to the YAML file reduces the time it takes to understand them and mitigates the risk of system failure.
Collaborate With Others
Adding comments allows team members to easily understand and modify code created by others. This helps in making collaborative development faster and more productive.
For instance, assume you have a Kubernetes deployment file for a blog service that looks like this:
apiVersion: apps/v1
kind: Deployment
metadata:
name: blogapp
…
# Temporarily use raw values for setting database
# credentials as environment variables,
# should use secret management tools like Vault to store these secrets
env:
- name: POSTGRES_DB
value: blog
- name: POSTGRES_USER
value: blog
- name: POSTGRES_PASSWORD
value: blog
- name: POSTGRES_HOST
value: postgresql:5432
ports:
- containerPort: 8081
The deployment script uses raw values for database credentials, which is not secure. If a malicious user were to access the deployment file, they could manipulate your PostgreSQL database. You know that you are supposed to use a secret management tool like Vault to store the database secrets, but because you’re in a hurry, you temporarily use this approach. Adding comments to the deployment script will remind you or others to update the script and use a secret management tool to store secret values.
Deactivate Certain Parts of the Script
Another benefit of adding comments is to deactivate certain parts of the script in YAML so that you can test whether your solution works or not.
Below is an example of a docker-compose.yml file for deploying a backend service, along with PostgreSQL and Redis databases.
version: "3.8"
services:
backend:
image: medusa-backend:latest
container_name: medusa-backend
restart: always
depends_on:
- postgres
- redis
environment:
DATABASE_URL: postgres://donald:le@postgres-medusa:5432/medusa-store
REDIS_URL: redis://redis-medusa:6379
JWT_SECRET: something
COOKIE_SECRET: something
DATABASE_TYPE: postgres
ports:
- "9000:9000"
# networks:
# - medusa
postgres:
image: postgres:latest
container_name: postgres-medusa
environment:
POSTGRES_USER: donald
POSTGRES_PASSWORD: le
POSTGRES_DB: medusa-store
ports:
- "5432:5432"
# networks:
# - medusa
redis:
image: redis:latest
container_name: redis-medusa
restart: always
ports:
- "6379:6379"
# networks:
# - medusa
#networks:
# medusa:
# name: medusa
Here the developer is not sure whether they need to define the Docker containers using the same network named medusa for these containers to communicate with each other. Thus, they comment on the definition of network block and the usage of the medusa network in each container.
After commenting on the usage of networks and trying to bring up all the containers, the Docker containers are still able to communicate with each other. Now they can safely remove the commented code. Below is the final optimized YAML code to deploy the Docker containers:
version: "3.8"
services:
backend:
image: medusa-backend:latest
container_name: medusa-backend
restart: always
depends_on:
- postgres
- redis
environment:
DATABASE_URL: postgres://donald:le@postgres-medusa:5432/medusa-store
REDIS_URL: redis://redis-medusa:6379
JWT_SECRET: something
COOKIE_SECRET: something
DATABASE_TYPE: postgres
ports:
- "9000:9000"
postgres:
image: postgres:latest
container_name: postgres-medusa
environment:
POSTGRES_USER: donald
POSTGRES_PASSWORD: le
POSTGRES_DB: medusa-store
ports:
- "5432:5432"
redis:
image: redis:latest
container_name: redis-medusa
restart: always
ports:
- "6379:6379"
You have just gone through some practical examples illustrating how to use comments in your YAML code. Let’s now see some of the best practices to follow when using comments in YAML.
When and Where To Use Comments in YAML
Some of the benefits of writing comments in YAML include:
- Allows us to explain difficult concepts
- Allows us to indicate the limitations of the current code
- Allows us to include additional code context
However, adding too many comments in a YAML file can be verbose and confusing. You should only write comments when needed. Below are some of the best practices to follow when working with comments in YAML.
Write the YAML block comments if you want to:
- Gives a summary of what the YAML file will do
- Explain a large block of code
Write the YAML in-line comment if you need to:
- Provides a brief explanation
- Explain an element or an object of the YAML code
Conclusion
Now you know how and when to write comments in YAML. You also know how to handle multiple-line comments and the best practices to follow when working with comments. Note that although comments make your script readable, they can clutter it when misused.
The tips we shared here give you a few ideas for getting started with YAML comments.
To explore more practical use cases of YAML, check out our Kubernetes For Beginners course.